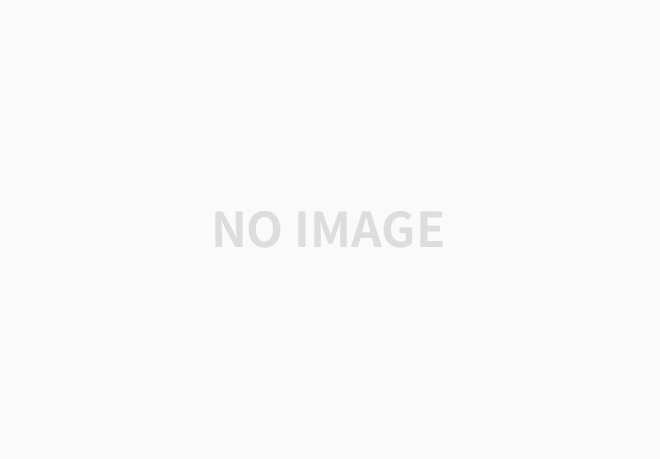
TensorFlow Lite 변환/build에 관한 전체 총정리 링크: 2020/04/05 - [TensorFlow] - TensorFlow Lite 사용하는법!
TensorFlow Lite를 사용하려면 일단 기존의 모델을 TensorFlow Lite 모델로 변환해서 사용해야 합니다.
TensorFlow Lite가 아직 최신기술(?)이다 보니, 구 버전의 TensorFlow(1.X)나 통합 이전의 keras를 이용해 만든 모델은 tflite 변환이 안되거나, 되더라도 Quantization 같은 최신 TensorFlow Lite 기능은 사용할 수 없습니다.
여기서는 어떻게 기존의 모델을 TensorFlow Lite 모델로 변환하는지 소개해 드리겠습니다.
TensorFlow 2.0 ~
SavedModel 에서 변환하기
import tensorflow as tf
export_dir = "model/my_saved_model"
converter = tf.lite.TFLiteConverter.from_saved_model(export_dir)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
Keras 에서 변환하기
import tensorflow as tf
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
concrete function 에서 변환하기
import tensorflow as tf
converter = tf.lite.TFLiteConverter.from_concrete_functions([concrete_func])
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
추가 옵션
- 양자화 (Quantization)
기법 샘플 데이터 요구 사이즈 감소 정확도 하드웨어 지원 Post-training float16 quantization 아니요 최대 50% Insignificant accuracy loss CPU, GPU Post-training dynamic range quantization 아니요 최대 75% Accuracy loss CPU Post-training integer quantization Unlabelled representative sample 최대 75% Smaller accuracy loss CPU, EdgeTPU, Hexagon DSP Quantization-aware training Labelled training data 최대 75% Smallest accuracy loss CPU, EdgeTPU, Hexagon DSP
Dynamic range quantization
import tensorflow as tf # 아무 방식으로나 불러와도 됩니다. converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir) converter.optimizations = [tf.lite.Optimize.DEFAULT] tflite_quant_model = converter.convert()
Full integer quantization of weights and activations
import tensorflow as tf def representative_dataset_gen(): for _ in range(num_calibration_steps): # 원하는 방식대로 샘플 데이터를 numpy array로 반환 yield [input] converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir) converter.optimizations = [tf.lite.Optimize.DEFAULT] converter.representative_dataset = representative_dataset_gen tflite_quant_model = converter.convert()
이렇게 해도 input / output Tensor들은 편의성을 위해 Float으로 유지가 된다고 합니다.
이것마저도 int로 양자화 한다면 변환하기 전에 다음 명령어를 추가하면 됩니다. (연산자에 따라 안될 수 도 있음)converter.target_spec.supported_ops = [tf.lite.OpsSet.TFLITE_BUILTINS_INT8] converter.inference_input_type = tf.uint8 converter.inference_output_type = tf.uint8
Float16 quantization of weights
import tensorflow as tf converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir) converter.optimizations = [tf.lite.Optimize.DEFAULT] converter.target_spec.supported_types = [tf.lite.constants.FLOAT16] tflite_quant_model = converter.convert()
TensorFlow 1.15
import tensorflow as tf
# Converting a GraphDef from session.
converter = tf.lite.TFLiteConverter.from_session(sess, in_tensors, out_tensors)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
# Converting a GraphDef from file.
converter = tf.lite.TFLiteConverter.from_frozen_graph(
graph_def_file, input_arrays, output_arrays)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
# Converting a SavedModel.
converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
# Converting a tf.keras model.
converter = tf.lite.TFLiteConverter.from_keras_model_file(keras_model)
tflite_model = converter.convert()
open("converted_model.tflite", "wb").write(tflite_model)
TensorFlow 1.12
※ TensorFlow Lite가 아직 contrib 일때 입니다. Quantization 사용 불가.
import tensorflow as tf
converter = tf.contrib.lite.TFLiteConverter.from_session(sess, in_tensors, out_tensors)
tflite_model = converter.convert()
import tensorflow as tf
converter = tf.contrib.lite.TFLiteConverter.from_frozen_graph(graph_def_file, input_arrays, output_arrays)
tflite_model = converter.convert()
import tensorflow as tf
converter = tf.contrib.lite.TFLiteConverter.from_saved_model(saved_model_dir)
tflite_model = converter.convert()
import tensorflow as tf
# keras_model은 저장된 파일 위치입니다. 아직 이버전에서는 바로 불러오기가 안됩니다.
converter = tf.contrib.lite.TFLiteConverter.from_keras_model_file(keras_model)
tflite_model = converter.convert()
사실 저도 2.0 이전 구 버전 들은 다 해본게 아니라서, 해당 버전들은 위의 링크나 GitHub에서 branch로 버전 골라서 소스파일 바로 확인하시면 예시가 조금 적혀있습니다.
공식 페이지: https://www.tensorflow.org/lite/convert/python_api
Converter Python API guide | TensorFlow Lite
This page provides examples on how to use the TensorFlow Lite converter using the Python API. Note: This only contains documentation on the Python API in TensorFlow 2. Documentation on using the Python API in TensorFlow 1 is available on GitHub. Python API
www.tensorflow.org
버전별 API: https://www.tensorflow.org/versions
TensorFlow API Versions
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License, and code samples are licensed under the Apache 2.0 License. For details, see the Google Developers Site Policies. Java is a registered trade
www.tensorflow.org
TensorFlow 버전별 Lite 문서
2.2: https://www.tensorflow.org/versions/r2.2/api_docs/python/tf/lite/TFLiteConverter
2.1: https://www.tensorflow.org/versions/r2.0/api_docs/python/tf/lite/TFLiteConverter
2.0: https://www.tensorflow.org/versions/r2.0/api_docs/python/tf/lite/TFLiteConverter
1.15: https://github.com/tensorflow/docs/blob/r1.15/site/en/api_docs/python/tf/lite/TFLiteConverter.md
1.14:https://github.com/tensorflow/docs/blob/r1.14/site/en/api_docs/python/tf/lite/TFLiteConverter.md
1.13: https://github.com/tensorflow/docs/blob/r1.13/site/en/api_docs/python/tf/lite/TFLiteConverter.md
'TensorFlow > Tensorflow Lite' 카테고리의 다른 글
Tensorflow Lite 실행하기(PC/C++) (2) | 2021.08.30 |
---|---|
Tensorflow Lite 를 C/C++로 빌드하기(bazel) (1) | 2021.08.29 |
Android 에서 TensorFlow Lite 사용하기 (C/C++) - ( 2/2 ) (9) | 2020.07.27 |
Android 에서 TensorFlow Lite 사용하기 (C/C++) - ( 1/2 ) (0) | 2020.04.05 |
TensorFlow Lite 빌드하기(bazel & C/C++) (0) | 2020.04.05 |